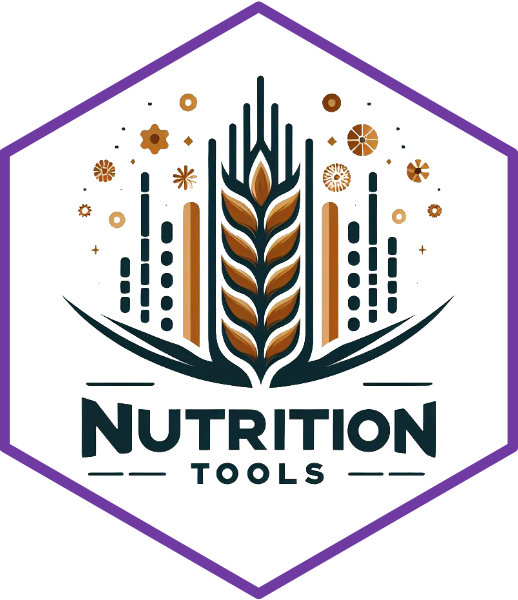
ENERCKcal calculator A function used to calculate Energy values in kcal
Source:R/ENERCKcal_calculator.R
ENERCKcal_calculator.Rd
This function has two modes. Either it works as a basic calculator - The values for Total Protein in grams, Total Fat in grams (ideally combined), Available Carbohydrate in grams, Fibre, Total Dietary in grams and Alcohol in grams are combined to find the Energy in kcal.
Alternatively, this function works as a data.frame calculator. If the
'df'
input is used, then the Nutrient inputs should be the names of
the columns in 'df'
which contains the details of their namesakes.
The result of this function will be a new column in df with the
ENERCKcal_calculated values.
Usage
ENERCKcal_calculator(
Protein = "PROCNTg",
Fat = "FATg",
Carbohydrate = "CHOAVLg",
Fibre = "FIBTGg",
Alcohol = "ALCg",
df,
comment_col = "comments",
Stop_If_Missing = TRUE,
Assume_Zero_Alcohol = FALSE,
Show_NA_outputs = TRUE
)
Arguments
- Protein
Required - default:
"PROCNTg"
- If using'df'
, then this should be the name of the column that contains Protein values in grams. If NOT using'df'
, this should be the number or list of numbers representing Protein values you are looking to calculate an Energy value using.- Fat
Required - default:
"FATg"
- If using'df'
, then this should be the name of the column that contains Total Fat values in grams. If NOT using'df'
, this should be the number or list of numbers representing Fat values you are looking to calculate an Energy value using.- Carbohydrate
Required - default:
"CHOAVLg"
- If using'df'
, then this should be the name of the column that contains Available Carbohydrate values in grams. If NOT using'df'
, this should be the number or list of numbers representing Available Carbohydrate values you are looking to calculate an Energy value using.- Fibre
Required - default:
"FIBTGg"
- If using'df'
, then this should be the name of the column that contains Fibre values in grams. If NOT using'df'
, this should be the number or list of numbers representing Fibre values you are looking to calculate an Energy value using.- Alcohol
Required - default:
"ALCg"
- If using'df'
, then this should be the name of the column that contains Alcohol values in grams. If NOT using'df'
, this should be the number or list of numbers representing Alcohol values you are looking to calculate an Energy value using. Note: This is the only Optional input. All items in all other nutrient parameters must have a valid value for the calculation - however, if Stop_If_Missing is set toFALSE
(as it is by default), then ALcohol values can contain blanks, which will be reset to 0.- df
Optional - If using on a data.frame, then this input should be the data.frame in question. If left blank, then the standard calculation will take place instead.
- comment_col
Optional - default:
"comments"
- only relevant if'df'
is in use. The name of the comment column in the df. If there isn't one present, then the function will create one to record the calculation details.- Stop_If_Missing
Required - default:
TRUE
- EitherTRUE
orFALSE
. If set toTRUE
, then the function will stop if it detects missing values in any of the columns or input values. If set toFALSE
, then the function will not stop if it detects missing values. Missing values will lead to NA ENERCKcal_calculated values.- Assume_Zero_Alcohol
Required - default:
FALSE
- EitherTRUE
orFALSE
. If set toTRUE
, and'Stop_If_Missing'
is set toFALSE
, then missing alcohol values will be set to 0, and a comment added if running the data.frame calculation. if set toFALSE
, then the Alcohol value will not change.- Show_NA_outputs
Required - default:
TRUE
- EitherTRUE
orFALSE
. If set toTRUE
then if any NA values are generated for ENERCKcal_calculated, a data.frame of these values will be shown.
Value
If using the data.frame input, the return will be a data.frame with a newly generated ENERCKcal_calculated column. If not using the data.frame input, then the return will be ENERCKcal_calculated values calculated from the input values put into the function.
Examples
# data.frame calculation: ----
# For this example, we will use the breakfast_df example dataset. However,
# that dataset has been designed to test a range of functions, and in its raw
# form is incompatable with ENERCKcal_calculator - therefore some changes will
# have to be made before it can be used.
# removing rows with many empty nutrient values
modified_breakfast_df <- breakfast_df[c(1:2, 4:8),]
modified_breakfast_df
#> food_code food_name WATERg PROCNTg FAT_g_combined CHOAVLg FIBTGg_combined
#> 1 F0001 Bacon 10 15 21 10 12
#> 2 F0002 Beans 15 10 12 1 3
#> 4 F0004 Mushroom 25 15 16 46 15
#> 5 F0005 Eggs 30 21 11 20 6
#> 6 F0006 Tomato 35 28 33 2 2
#> 7 F0007 Sausage 40 10 13 24 9
#> 8 F0008 Butter NA 27 16 22 13
#> ALCg ASHg THIAmg THIAHCLmg RETOLmcg CARTBEQmcg_combined NIAmg TRPmg NIAEQmg
#> 1 12 12 32 21 53 NA 172.0 126.0 85.3
#> 2 43 3 90 45 12 51 NA 142.5 49.2
#> 4 15 15 21 69 NA 22 NA 167.0 23.2
#> 5 6 6 <NA> 42 62 62 8.1 NA 30.5
#> 6 2 2 61 150 40 102 134.6 76.3 83.3
#> 7 9 9 21 23 140 32 10.2 98.6 16.6
#> 8 13 13 30 210 72 187.9 41.4 84.5
#> NIATRPmg FATg FAT_g FATCEg comments
#> 1 2.10 21 20.9 NA
#> 2 NA NA 12.0 NA These are imaginary food items
#> 4 2.80 NA NA 16.0 With imaginary nutrient values
#> 5 NA 11 10.9 NA
#> 6 1.27 NA 33.0 33.0 And blanks
#> 7 1.64 13 12.1 NA <NA>
#> 8 0.69 16 16.1 15.9 To test different outputs
modified_breakfast_df <- ENERCKcal_calculator(
df = modified_breakfast_df,
Protein = "PROCNTg",
Fat = "FAT_g_combined",
Carbohydrate = "CHOAVLg",
Fibre = "FIBTGg_combined",
Alcohol = "ALCg"
)
modified_breakfast_df
#> food_code food_name WATERg PROCNTg FAT_g_combined CHOAVLg FIBTGg_combined
#> 1 F0001 Bacon 10 15 21 10 12
#> 2 F0002 Beans 15 10 12 1 3
#> 4 F0004 Mushroom 25 15 16 46 15
#> 5 F0005 Eggs 30 21 11 20 6
#> 6 F0006 Tomato 35 28 33 2 2
#> 7 F0007 Sausage 40 10 13 24 9
#> 8 F0008 Butter NA 27 16 22 13
#> ALCg ASHg THIAmg THIAHCLmg RETOLmcg CARTBEQmcg_combined NIAmg TRPmg NIAEQmg
#> 1 12 12 32 21 53 NA 172.0 126.0 85.3
#> 2 43 3 90 45 12 51 NA 142.5 49.2
#> 4 15 15 21 69 NA 22 NA 167.0 23.2
#> 5 6 6 <NA> 42 62 62 8.1 NA 30.5
#> 6 2 2 61 150 40 102 134.6 76.3 83.3
#> 7 9 9 21 23 140 32 10.2 98.6 16.6
#> 8 13 13 30 210 72 187.9 41.4 84.5
#> NIATRPmg FATg FAT_g FATCEg
#> 1 2.10 21 20.9 NA
#> 2 NA NA 12.0 NA
#> 4 2.80 NA NA 16.0
#> 5 NA 11 10.9 NA
#> 6 1.27 NA 33.0 33.0
#> 7 1.64 13 12.1 NA
#> 8 0.69 16 16.1 15.9
#> comments
#> 1 ENERCKcal_calculated calculated to be 397 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 2 These are imaginary food items; ENERCKcal_calculated calculated to be 459 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 4 With imaginary nutrient values; ENERCKcal_calculated calculated to be 523 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 5 ENERCKcal_calculated calculated to be 317 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 6 And blanks; ENERCKcal_calculated calculated to be 435 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 7 ENERCKcal_calculated calculated to be 334 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 8 To test different outputs; ENERCKcal_calculated calculated to be 457 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> ENERCKcal_calculated
#> 1 397
#> 2 459
#> 4 523
#> 5 317
#> 6 435
#> 7 334
#> 8 457
# However, if some of the options are changed, the function can accept any
# input - however, this is not advised. This will result in only
# partial coverage of the data.frame, as the things that stopped the function
# running before will lead to NA results now.
# Before:
breakfast_df_1 <- ENERCKcal_calculator(
df = breakfast_df,
Protein = "PROCNTg",
Fat = "FAT_g_combined",
Carbohydrate = "CHOAVLg",
Fibre = "FIBTGg_combined",
Alcohol = "ALCg"
)
#> Missing alcohol values detected. Please fill in the missing alcohol values, and try again.
#>
#> Missing alcohol values returned as df.
#> food_code food_name WATERg PROCNTg FAT_g_combined CHOAVLg
#> 9 F0009 Brown Sauce NA NA NA NA
#> 10 F0010 Tomato Ketchup NA NA NA NA
#> FIBTGg_combined ALCg ASHg THIAmg THIAHCLmg RETOLmcg CARTBEQmcg_combined
#> 9 NA NA NA 59 52 41 112
#> 10 NA NA NA <NA> NA NA NA
#> NIAmg TRPmg NIAEQmg NIATRPmg FATg FAT_g FATCEg comments TEMPnewcomment
#> 9 92 172 17.7 2.87 NA NA NA NA
#> 10 NA NA NA NA NA NA NA And scenarios NA
breakfast_df_1
#> food_code food_name WATERg PROCNTg FAT_g_combined CHOAVLg
#> 9 F0009 Brown Sauce NA NA NA NA
#> 10 F0010 Tomato Ketchup NA NA NA NA
#> FIBTGg_combined ALCg ASHg THIAmg THIAHCLmg RETOLmcg CARTBEQmcg_combined
#> 9 NA NA NA 59 52 41 112
#> 10 NA NA NA <NA> NA NA NA
#> NIAmg TRPmg NIAEQmg NIATRPmg FATg FAT_g FATCEg comments TEMPnewcomment
#> 9 92 172 17.7 2.87 NA NA NA NA
#> 10 NA NA NA NA NA NA NA And scenarios NA
# In this case, the missing Alcohol values are preventing the function
# running. They have been returned as the df, to allow you to fix these issues
# and then run it again, and to allow you to see where this issue is occurring.
# After:
breakfast_df_2 <- ENERCKcal_calculator(
df = breakfast_df,
Protein = "PROCNTg",
Fat = "FAT_g_combined",
Carbohydrate = "CHOAVLg",
Fibre = "FIBTGg_combined",
Alcohol = "ALCg",
Stop_If_Missing = FALSE
)
#> NA output values found. Viewing ENERCKcal_calculated values of NA.
#> food_code food_name WATERg PROCNTg FAT_g_combined CHOAVLg
#> 3 F0003 Toast 20 20 NA 24
#> 9 F0009 Brown Sauce NA NA NA NA
#> 10 F0010 Tomato Ketchup NA NA NA NA
#> FIBTGg_combined ALCg ASHg THIAmg THIAHCLmg RETOLmcg CARTBEQmcg_combined
#> 3 8 8 28 130 20 20 91
#> 9 NA NA NA 59 52 41 112
#> 10 NA NA NA <NA> NA NA NA
#> NIAmg TRPmg NIAEQmg NIATRPmg FATg FAT_g FATCEg
#> 3 24.4 142.7 86.4 2.38 NA NA NA
#> 9 92.0 172.0 17.7 2.87 NA NA NA
#> 10 NA NA NA NA NA NA NA
#> comments
#> 3 ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 9 ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 10 And scenarios; ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> ENERCKcal_calculated
#> 3 NA
#> 9 NA
#> 10 NA
breakfast_df_2
#> food_code food_name WATERg PROCNTg FAT_g_combined CHOAVLg
#> 1 F0001 Bacon 10 15 21 10
#> 2 F0002 Beans 15 10 12 1
#> 3 F0003 Toast 20 20 NA 24
#> 4 F0004 Mushroom 25 15 16 46
#> 5 F0005 Eggs 30 21 11 20
#> 6 F0006 Tomato 35 28 33 2
#> 7 F0007 Sausage 40 10 13 24
#> 8 F0008 Butter NA 27 16 22
#> 9 F0009 Brown Sauce NA NA NA NA
#> 10 F0010 Tomato Ketchup NA NA NA NA
#> FIBTGg_combined ALCg ASHg THIAmg THIAHCLmg RETOLmcg CARTBEQmcg_combined
#> 1 12 12 12 32 21 53 NA
#> 2 3 43 3 90 45 12 51
#> 3 8 8 28 130 20 20 91
#> 4 15 15 15 21 69 NA 22
#> 5 6 6 6 <NA> 42 62 62
#> 6 2 2 2 61 150 40 102
#> 7 9 9 9 21 23 140 32
#> 8 13 13 13 30 210 72
#> 9 NA NA NA 59 52 41 112
#> 10 NA NA NA <NA> NA NA NA
#> NIAmg TRPmg NIAEQmg NIATRPmg FATg FAT_g FATCEg
#> 1 172.0 126.0 85.3 2.10 21 20.9 NA
#> 2 NA 142.5 49.2 NA NA 12.0 NA
#> 3 24.4 142.7 86.4 2.38 NA NA NA
#> 4 NA 167.0 23.2 2.80 NA NA 16.0
#> 5 8.1 NA 30.5 NA 11 10.9 NA
#> 6 134.6 76.3 83.3 1.27 NA 33.0 33.0
#> 7 10.2 98.6 16.6 1.64 13 12.1 NA
#> 8 187.9 41.4 84.5 0.69 16 16.1 15.9
#> 9 92.0 172.0 17.7 2.87 NA NA NA
#> 10 NA NA NA NA NA NA NA
#> comments
#> 1 ENERCKcal_calculated calculated to be 397 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 2 These are imaginary food items; ENERCKcal_calculated calculated to be 459 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 3 ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 4 With imaginary nutrient values; ENERCKcal_calculated calculated to be 523 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 5 ENERCKcal_calculated calculated to be 317 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 6 And blanks; ENERCKcal_calculated calculated to be 435 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 7 ENERCKcal_calculated calculated to be 334 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 8 To test different outputs; ENERCKcal_calculated calculated to be 457 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 9 ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 10 And scenarios; ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> ENERCKcal_calculated
#> 1 397
#> 2 459
#> 3 NA
#> 4 523
#> 5 317
#> 6 435
#> 7 334
#> 8 457
#> 9 NA
#> 10 NA
# There are NA values for where Alcohol is missing, and where Fat is missing.
# This can be further modified, however. If there are missing Alcohol values,
# but the rest of the values are there, it is possible to set the function to
# set missing alcohol values to 0, through Stop_If_Missing = FALSE and
# Assume_Zero_Alcohol = TRUE.
modified_breakfast_df_2 <- breakfast_df
modified_breakfast_df_2[5, "ALCg"] <- NA
# Here we have artificially created this situation. When running the function
# with only Stop_If_Missing = FALSE, this is the result:
modified_breakfast_df_2_output_1 <- ENERCKcal_calculator(
df = modified_breakfast_df_2,
Protein = "PROCNTg",
Fat = "FAT_g_combined",
Carbohydrate = "CHOAVLg",
Fibre = "FIBTGg_combined",
Alcohol = "ALCg",
Stop_If_Missing = FALSE
)
#> NA output values found. Viewing ENERCKcal_calculated values of NA.
#> food_code food_name WATERg PROCNTg FAT_g_combined CHOAVLg
#> 3 F0003 Toast 20 20 NA 24
#> 5 F0005 Eggs 30 21 11 20
#> 9 F0009 Brown Sauce NA NA NA NA
#> 10 F0010 Tomato Ketchup NA NA NA NA
#> FIBTGg_combined ALCg ASHg THIAmg THIAHCLmg RETOLmcg CARTBEQmcg_combined
#> 3 8 8 28 130 20 20 91
#> 5 6 NA 6 <NA> 42 62 62
#> 9 NA NA NA 59 52 41 112
#> 10 NA NA NA <NA> NA NA NA
#> NIAmg TRPmg NIAEQmg NIATRPmg FATg FAT_g FATCEg
#> 3 24.4 142.7 86.4 2.38 NA NA NA
#> 5 8.1 NA 30.5 NA 11 10.9 NA
#> 9 92.0 172.0 17.7 2.87 NA NA NA
#> 10 NA NA NA NA NA NA NA
#> comments
#> 3 ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 5 ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 9 ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 10 And scenarios; ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> ENERCKcal_calculated
#> 3 NA
#> 5 NA
#> 9 NA
#> 10 NA
modified_breakfast_df_2_output_1
#> food_code food_name WATERg PROCNTg FAT_g_combined CHOAVLg
#> 1 F0001 Bacon 10 15 21 10
#> 2 F0002 Beans 15 10 12 1
#> 3 F0003 Toast 20 20 NA 24
#> 4 F0004 Mushroom 25 15 16 46
#> 5 F0005 Eggs 30 21 11 20
#> 6 F0006 Tomato 35 28 33 2
#> 7 F0007 Sausage 40 10 13 24
#> 8 F0008 Butter NA 27 16 22
#> 9 F0009 Brown Sauce NA NA NA NA
#> 10 F0010 Tomato Ketchup NA NA NA NA
#> FIBTGg_combined ALCg ASHg THIAmg THIAHCLmg RETOLmcg CARTBEQmcg_combined
#> 1 12 12 12 32 21 53 NA
#> 2 3 43 3 90 45 12 51
#> 3 8 8 28 130 20 20 91
#> 4 15 15 15 21 69 NA 22
#> 5 6 NA 6 <NA> 42 62 62
#> 6 2 2 2 61 150 40 102
#> 7 9 9 9 21 23 140 32
#> 8 13 13 13 30 210 72
#> 9 NA NA NA 59 52 41 112
#> 10 NA NA NA <NA> NA NA NA
#> NIAmg TRPmg NIAEQmg NIATRPmg FATg FAT_g FATCEg
#> 1 172.0 126.0 85.3 2.10 21 20.9 NA
#> 2 NA 142.5 49.2 NA NA 12.0 NA
#> 3 24.4 142.7 86.4 2.38 NA NA NA
#> 4 NA 167.0 23.2 2.80 NA NA 16.0
#> 5 8.1 NA 30.5 NA 11 10.9 NA
#> 6 134.6 76.3 83.3 1.27 NA 33.0 33.0
#> 7 10.2 98.6 16.6 1.64 13 12.1 NA
#> 8 187.9 41.4 84.5 0.69 16 16.1 15.9
#> 9 92.0 172.0 17.7 2.87 NA NA NA
#> 10 NA NA NA NA NA NA NA
#> comments
#> 1 ENERCKcal_calculated calculated to be 397 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 2 These are imaginary food items; ENERCKcal_calculated calculated to be 459 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 3 ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 4 With imaginary nutrient values; ENERCKcal_calculated calculated to be 523 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 5 ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 6 And blanks; ENERCKcal_calculated calculated to be 435 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 7 ENERCKcal_calculated calculated to be 334 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 8 To test different outputs; ENERCKcal_calculated calculated to be 457 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 9 ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 10 And scenarios; ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> ENERCKcal_calculated
#> 1 397
#> 2 459
#> 3 NA
#> 4 523
#> 5 NA
#> 6 435
#> 7 334
#> 8 457
#> 9 NA
#> 10 NA
# However, if we change the other option as well:
modified_breakfast_df_2_output_2 <- ENERCKcal_calculator(
df = modified_breakfast_df_2,
Protein = "PROCNTg",
Fat = "FAT_g_combined",
Carbohydrate = "CHOAVLg",
Fibre = "FIBTGg_combined",
Alcohol = "ALCg",
Stop_If_Missing = FALSE,
Assume_Zero_Alcohol = TRUE
)
#> NA output values found. Viewing ENERCKcal_calculated values of NA.
#> food_code food_name WATERg PROCNTg FAT_g_combined CHOAVLg
#> 3 F0003 Toast 20 20 NA 24
#> 9 F0009 Brown Sauce NA NA NA NA
#> 10 F0010 Tomato Ketchup NA NA NA NA
#> FIBTGg_combined ALCg ASHg THIAmg THIAHCLmg RETOLmcg CARTBEQmcg_combined
#> 3 8 8 28 130 20 20 91
#> 9 NA 0 NA 59 52 41 112
#> 10 NA 0 NA <NA> NA NA NA
#> NIAmg TRPmg NIAEQmg NIATRPmg FATg FAT_g FATCEg
#> 3 24.4 142.7 86.4 2.38 NA NA NA
#> 9 92.0 172.0 17.7 2.87 NA NA NA
#> 10 NA NA NA NA NA NA NA
#> comments
#> 3 ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 9 Missing Alcohol value set to 0; ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 10 And scenarios; Missing Alcohol value set to 0; ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> ENERCKcal_calculated
#> 3 NA
#> 9 NA
#> 10 NA
modified_breakfast_df_2_output_2
#> food_code food_name WATERg PROCNTg FAT_g_combined CHOAVLg
#> 1 F0001 Bacon 10 15 21 10
#> 2 F0002 Beans 15 10 12 1
#> 3 F0003 Toast 20 20 NA 24
#> 4 F0004 Mushroom 25 15 16 46
#> 5 F0005 Eggs 30 21 11 20
#> 6 F0006 Tomato 35 28 33 2
#> 7 F0007 Sausage 40 10 13 24
#> 8 F0008 Butter NA 27 16 22
#> 9 F0009 Brown Sauce NA NA NA NA
#> 10 F0010 Tomato Ketchup NA NA NA NA
#> FIBTGg_combined ALCg ASHg THIAmg THIAHCLmg RETOLmcg CARTBEQmcg_combined
#> 1 12 12 12 32 21 53 NA
#> 2 3 43 3 90 45 12 51
#> 3 8 8 28 130 20 20 91
#> 4 15 15 15 21 69 NA 22
#> 5 6 0 6 <NA> 42 62 62
#> 6 2 2 2 61 150 40 102
#> 7 9 9 9 21 23 140 32
#> 8 13 13 13 30 210 72
#> 9 NA 0 NA 59 52 41 112
#> 10 NA 0 NA <NA> NA NA NA
#> NIAmg TRPmg NIAEQmg NIATRPmg FATg FAT_g FATCEg
#> 1 172.0 126.0 85.3 2.10 21 20.9 NA
#> 2 NA 142.5 49.2 NA NA 12.0 NA
#> 3 24.4 142.7 86.4 2.38 NA NA NA
#> 4 NA 167.0 23.2 2.80 NA NA 16.0
#> 5 8.1 NA 30.5 NA 11 10.9 NA
#> 6 134.6 76.3 83.3 1.27 NA 33.0 33.0
#> 7 10.2 98.6 16.6 1.64 13 12.1 NA
#> 8 187.9 41.4 84.5 0.69 16 16.1 15.9
#> 9 92.0 172.0 17.7 2.87 NA NA NA
#> 10 NA NA NA NA NA NA NA
#> comments
#> 1 ENERCKcal_calculated calculated to be 397 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 2 These are imaginary food items; ENERCKcal_calculated calculated to be 459 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 3 ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 4 With imaginary nutrient values; ENERCKcal_calculated calculated to be 523 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 5 Missing Alcohol value set to 0; ENERCKcal_calculated calculated to be 275 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 6 And blanks; ENERCKcal_calculated calculated to be 435 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 7 ENERCKcal_calculated calculated to be 334 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 8 To test different outputs; ENERCKcal_calculated calculated to be 457 through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 9 Missing Alcohol value set to 0; ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> 10 And scenarios; Missing Alcohol value set to 0; ENERCKcal_calculated calculated to be NA through PROCNTg*4 + FAT_g_combined*9 + CHOAVLg*4 + FIBTGg_combined*2 + ALCg*7
#> ENERCKcal_calculated
#> 1 397
#> 2 459
#> 3 NA
#> 4 523
#> 5 275
#> 6 435
#> 7 334
#> 8 457
#> 9 NA
#> 10 NA
# We can see that the function has run, and the row with the missing Alcohol
# value has been able to generate a result - as well as having its ALcohol
# value changed. An additional comment has been added to record this change.
#Single calculation: ----
#Bread, wheat, white, unfortified
Protein_value <- 7.5
Fat_value <- 1.3
Carb_value <- 50.5
Fibre_value <- 2.9
Alcohol_value <- 0
ENERCKcal_calculated <- ENERCKcal_calculator(Protein = Protein_value, Fat =
Fat_value, Carbohydrate = Carb_value, Fibre = Fibre_value, Alcohol =
Alcohol_value)
ENERCKcal_calculated
#> [1] 249.5
#alternatively:
ENERCKcal_calculated_2 <- ENERCKcal_calculator(7.5, 1.3, 50.5, 2.9, 0)
ENERCKcal_calculated_2
#> [1] 249.5
# Or, for multiple values (secondary values are fictional):
ENERCKcal_calculated_3 <- ENERCKcal_calculator(c(7.5, 5), c(1.3, 2), c(50.5,
51.5), c(2.9, 5), c(0, 1))
ENERCKcal_calculated_3
#> [1] 249.5 261.0
# However, if there are blank values, then the function will not work
ENERCKcal_calculated_4 <- ENERCKcal_calculator(c(7.5, 5), c(1.3, 2), c(50.5,
51.5), c(2.9, 5), c(0, NA))
#> NA/'' detected in Alcohol. Stopping. Please ensure all items have Alcohol values.
# Unless other options are selected - such as Stop_If_Missing is turned off
ENERCKcal_calculated_5 <- ENERCKcal_calculator(c(7.5, 5), c(1.3, 2), c(50.5,
51.5), c(2.9, 5), c(0, NA), Stop_If_Missing = FALSE)
ENERCKcal_calculated_5
#> [1] 249.5 NA
# In this format we can apply Assume_Zero_Alcohol as well
ENERCKcal_calculated_6 <- ENERCKcal_calculator(c(7.5, 5), c(1.3, 2), c(50.5,
51.5), c(2.9, 5), c(0, NA), Stop_If_Missing = FALSE, Assume_Zero_Alcohol =
TRUE)
ENERCKcal_calculated_6
#> [1] 249.5 254.0