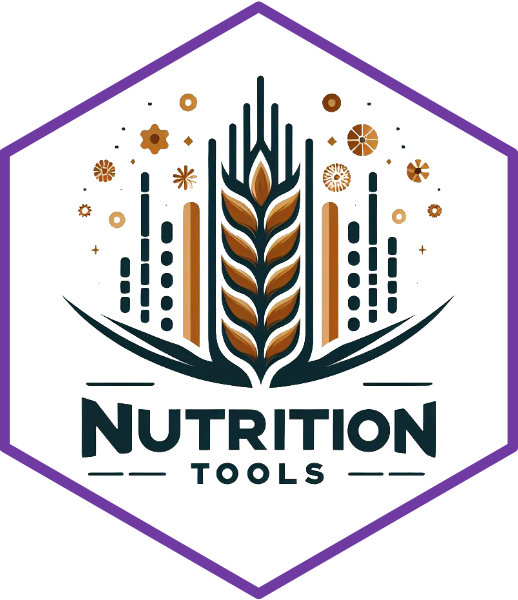
Carbohydrates (calculated by difference) Calculator
Source:R/CHOAVLDFg_calculator.R
CHOAVLDFg_calculator.Rd
Calculates CHOAVLDFg_calculated = (100 - (WATERg + PROTg + FATg_combined + FBGTg + ASHg + ALCg)). Column names are case sensitive and an error is returned if not found. Non-numeric columns will be converted if possible in function to be numeric.
Usage
CHOAVLDFg_calculator(
df,
WATERg_column = "WATERg",
PROCNTg_column = "PROCNTg",
FAT_g_combined_column = "FAT_g_combined",
FIBTGg_combined_column = "FIBTGg_combined",
ALCg_column = "ALCg",
ASHg_column = "ASHg",
comment = TRUE,
comment_col = "comments",
NegativeValueReplacement = 0,
NegativeValueDF = FALSE
)
Arguments
- df
Required - the data.frame the data is currently stored in.
- WATERg_column
Required - default:
'WATERg'
- The name of the column containing Water/moisture content in grams per 100g of Edible Portion (EP).- PROCNTg_column
Required - default:
'PROCNTg'
- Protein in grams per 100g of Edible Portion (EP), as reported in the original FCT and assumed to be calculated from nitrogen (NTg) content.- FAT_g_combined_column
Required - default:
'FAT_g_combined'
- Fat content, unknown method of calculation, in grams per 100g of Edible Portion (EP).- FIBTGg_combined_column
Required - default:
'FIBTGg_combined'
- Fibre content from combined Tagnames, with preference of Total dietary fibre by AOAC Prosky method, expressed in grams per 100g of Edible Portion (EP).- ALCg_column
Required - default:
'ALCg'
- Alcohol in grams per 100g of Edible Portion (EP).- ASHg_column
Required - default:
'ASHg'
- Ashes in grams per 100g of Edible Portion (EP).- comment
Required - default:
TRUE
-TRUE
orFALSE
. Ifcomment
is set toTRUE
(as it is by default), when the function is run a comment describing the source of theCHOAVLDFg_calculated
column is added to thecomment_col
If nocomment_col
is selected, andcomment = TRUE
, one is created.- comment_col
Optional - default:
'comments'
- A potential input variable; the column which contains the metadata comments for the food item in question. Not required ifcomment
is set toFALSE
. Ifcomment
is set toTRUE
, and thecomment_col
input is not the name of a column found in thedf
, the function will create a column with the name of thecomment_col
input to store comments in.- NegativeValueReplacement
Required - default:
0
- Options are0
,NA
,'remove'
, or'nothing'
. Choose what happens to negative values. If set to0
, then negative values are set to 0. If set toNA
, they are replaced with NA. if set to'remove'
, then those entries in thedf
are removed. if set to'nothing'
, then they are left as negative values.- NegativeValueDF
Required - default:
FALSE
-TRUE
orFALSE
. If set toTRUE
, Then the output switches from being a copy of the inputdf
with the theCHOAVLDFg_calculated
column to a subset of that dataframe only showingCHOAVLDFg_calculated
values that are less than 0, for manual inspection.
Value
Original data.frame with a new CHOAVLDFg_calculated
column, and
(depending on the options selected) an additional comment/comments column
and comment.
Examples
# Two example data.frames have been prepared to illustrate the
# CHOAVLDFg_calculator The first is a dataset of fictional food values to
# illustrate the various options in the function, and the second is a dataset
# with non-standard column names, to show how to specify columns.
# This is the first data.frame - before the CHOAVLDFg_calculator has been used on it.
breakfast_df <- breakfast_df[,c("food_code", "food_name", "WATERg",
"PROCNTg", "FAT_g_combined", "FIBTGg_combined", "ALCg", "ASHg",
"comments")]
breakfast_df
#> food_code food_name WATERg PROCNTg FAT_g_combined FIBTGg_combined ALCg
#> 1 F0001 Bacon 10 15 21 12 12
#> 2 F0002 Beans 15 10 12 3 43
#> 3 F0003 Toast 20 20 NA 8 8
#> 4 F0004 Mushroom 25 15 16 15 15
#> 5 F0005 Eggs 30 21 11 6 6
#> 6 F0006 Tomato 35 28 33 2 2
#> 7 F0007 Sausage 40 10 13 9 9
#> 8 F0008 Butter NA 27 16 13 13
#> 9 F0009 Brown Sauce NA NA NA NA NA
#> 10 F0010 Tomato Ketchup NA NA NA NA NA
#> ASHg comments
#> 1 12
#> 2 3 These are imaginary food items
#> 3 28 <NA>
#> 4 15 With imaginary nutrient values
#> 5 6
#> 6 2 And blanks
#> 7 9 <NA>
#> 8 13 To test different outputs
#> 9 NA
#> 10 NA And scenarios
#
#
# First, an example of the standard usecase - calculate the CHOAVLDFg_calculated
# value, while resetting negative values to 0.
NegativeToZeroResults <- CHOAVLDFg_calculator(breakfast_df, NegativeValueReplacement = 0)
#> ---------------------------
#>
#> 4 CHOAVLDFg_calculated values calculated to be less than 0. Minimum result: -2. Number of values below -5: 0. Number of NA results: 2. Please rerun the function with NegativeValueDF = TRUE if you wish to inspect these values.
#>
#> Negative values set to 0, as per user input.
#>
#> ---------------------------
#
NegativeToZeroResults
#> food_code food_name WATERg PROCNTg FAT_g_combined FIBTGg_combined ALCg
#> 1 F0001 Bacon 10 15 21 12 12
#> 2 F0002 Beans 15 10 12 3 43
#> 3 F0003 Toast 20 20 NA 8 8
#> 4 F0004 Mushroom 25 15 16 15 15
#> 5 F0005 Eggs 30 21 11 6 6
#> 6 F0006 Tomato 35 28 33 2 2
#> 7 F0007 Sausage 40 10 13 9 9
#> 8 F0008 Butter NA 27 16 13 13
#> 9 F0009 Brown Sauce NA NA NA NA NA
#> 10 F0010 Tomato Ketchup NA NA NA NA NA
#> ASHg
#> 1 12
#> 2 3
#> 3 28
#> 4 15
#> 5 6
#> 6 2
#> 7 9
#> 8 13
#> 9 NA
#> 10 NA
#> comments
#> 1 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 2 These are imaginary food items; CHOAVLDFg_calculated calculated from 100-[constituents]
#> 3 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 4 With imaginary nutrient values; CHOAVLDFg_calculated calculated from 100-[constituents] - Original value of -1 reset to 0
#> 5 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 6 And blanks; CHOAVLDFg_calculated calculated from 100-[constituents] - Original value of -2 reset to 0
#> 7 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 8 To test different outputs; CHOAVLDFg_calculated calculated from 100-[constituents]
#> 9
#> 10 And scenarios
#> CHOAVLDFg_calculated
#> 1 18
#> 2 14
#> 3 16
#> 4 0
#> 5 20
#> 6 0
#> 7 10
#> 8 18
#> 9 NA
#> 10 NA
# See the changes - the addition of the CHOAVLDFg_calculated column, and
# the additions to the comments column. Notice how some comments are
# specially formulated to show if the corresponding CHOAVLDFg_calculated
# value has been reset to 0.
#
#
# The second example shows the results when the Replacement option is set to NA
NegativeToNA_results <- CHOAVLDFg_calculator(breakfast_df, NegativeValueReplacement = NA)
#> ---------------------------
#>
#> 4 CHOAVLDFg_calculated values calculated to be less than 0. Minimum result: -2. Number of values below -5: 0. Number of NA results: 2. Please rerun the function with NegativeValueDF = TRUE if you wish to inspect these values.
#>
#> Negative values set to NA, as per user input.
#>
#> ---------------------------
#
NegativeToNA_results
#> food_code food_name WATERg PROCNTg FAT_g_combined FIBTGg_combined ALCg
#> 1 F0001 Bacon 10 15 21 12 12
#> 2 F0002 Beans 15 10 12 3 43
#> 3 F0003 Toast 20 20 NA 8 8
#> 4 F0004 Mushroom 25 15 16 15 15
#> 5 F0005 Eggs 30 21 11 6 6
#> 6 F0006 Tomato 35 28 33 2 2
#> 7 F0007 Sausage 40 10 13 9 9
#> 8 F0008 Butter NA 27 16 13 13
#> 9 F0009 Brown Sauce NA NA NA NA NA
#> 10 F0010 Tomato Ketchup NA NA NA NA NA
#> ASHg
#> 1 12
#> 2 3
#> 3 28
#> 4 15
#> 5 6
#> 6 2
#> 7 9
#> 8 13
#> 9 NA
#> 10 NA
#> comments
#> 1 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 2 These are imaginary food items; CHOAVLDFg_calculated calculated from 100-[constituents]
#> 3 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 4 With imaginary nutrient values; CHOAVLDFg_calculated calculated from 100-[constituents] - Original value of -1 reset to NA
#> 5 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 6 And blanks; CHOAVLDFg_calculated calculated from 100-[constituents] - Original value of -2 reset to NA
#> 7 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 8 To test different outputs; CHOAVLDFg_calculated calculated from 100-[constituents]
#> 9
#> 10 And scenarios
#> CHOAVLDFg_calculated
#> 1 18
#> 2 14
#> 3 16
#> 4 NA
#> 5 20
#> 6 NA
#> 7 10
#> 8 18
#> 9 NA
#> 10 NA
# Check the CHOAVLDFg_calculated column and comments column again - see how
# values below zero have been replaced with NA, and a note of this change
# logged in the comments column.
#
#
# The third example shows the results when the Replacement option is set to 'remove'
remove_results <- CHOAVLDFg_calculator(breakfast_df, NegativeValueReplacement = "remove")
#> ---------------------------
#>
#> 4 CHOAVLDFg_calculated values calculated to be less than 0. Minimum result: -2. Number of values below -5: 0. Number of NA results: 2. Please rerun the function with NegativeValueDF = TRUE if you wish to inspect these values.
#>
#> Negative value rows removed, as per user input.
#>
#> ---------------------------
#
remove_results
#> food_code food_name WATERg PROCNTg FAT_g_combined FIBTGg_combined ALCg ASHg
#> 1 F0001 Bacon 10 15 21 12 12 12
#> 2 F0002 Beans 15 10 12 3 43 3
#> 3 F0003 Toast 20 20 NA 8 8 28
#> 5 F0005 Eggs 30 21 11 6 6 6
#> 7 F0007 Sausage 40 10 13 9 9 9
#> 8 F0008 Butter NA 27 16 13 13 13
#> comments
#> 1 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 2 These are imaginary food items; CHOAVLDFg_calculated calculated from 100-[constituents]
#> 3 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 5 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 7 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 8 To test different outputs; CHOAVLDFg_calculated calculated from 100-[constituents]
#> CHOAVLDFg_calculated
#> 1 18
#> 2 14
#> 3 16
#> 5 20
#> 7 10
#> 8 18
# See how the out of bounds values have been removed.
#
#
# The fourth example is of the nothing results - i.e. nothing happens to the
# negative values.
NegativeNoChangeResults <- CHOAVLDFg_calculator(breakfast_df, NegativeValueReplacement = "nothing")
#> ---------------------------
#>
#> 4 CHOAVLDFg_calculated values calculated to be less than 0. Minimum result: -2. Number of values below -5: 0. Number of NA results: 2. Please rerun the function with NegativeValueDF = TRUE if you wish to inspect these values.
#>
#> Negative values left untouched, as per user input.
#>
#> ---------------------------
#
NegativeNoChangeResults
#> food_code food_name WATERg PROCNTg FAT_g_combined FIBTGg_combined ALCg
#> 1 F0001 Bacon 10 15 21 12 12
#> 2 F0002 Beans 15 10 12 3 43
#> 3 F0003 Toast 20 20 NA 8 8
#> 4 F0004 Mushroom 25 15 16 15 15
#> 5 F0005 Eggs 30 21 11 6 6
#> 6 F0006 Tomato 35 28 33 2 2
#> 7 F0007 Sausage 40 10 13 9 9
#> 8 F0008 Butter NA 27 16 13 13
#> 9 F0009 Brown Sauce NA NA NA NA NA
#> 10 F0010 Tomato Ketchup NA NA NA NA NA
#> ASHg
#> 1 12
#> 2 3
#> 3 28
#> 4 15
#> 5 6
#> 6 2
#> 7 9
#> 8 13
#> 9 NA
#> 10 NA
#> comments
#> 1 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 2 These are imaginary food items; CHOAVLDFg_calculated calculated from 100-[constituents]
#> 3 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 4 With imaginary nutrient values; CHOAVLDFg_calculated calculated from 100-[constituents]
#> 5 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 6 And blanks; CHOAVLDFg_calculated calculated from 100-[constituents]
#> 7 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 8 To test different outputs; CHOAVLDFg_calculated calculated from 100-[constituents]
#> 9 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 10 And scenarios
#> CHOAVLDFg_calculated
#> 1 18
#> 2 14
#> 3 16
#> 4 -1
#> 5 20
#> 6 -2
#> 7 10
#> 8 18
#> 9 NA
#> 10 NA
# Look at the CHOAVLDFg_calculator values - and see how they've been left,
# even if they're negative.
#
#
# The fifth example is of the negative dataframe - an option useful for identifying
# and examining negative results.
NegativeCarb_results <- CHOAVLDFg_calculator(breakfast_df, NegativeValueDF = TRUE)
#> ---------------------------
#>
#> 4 CHOAVLDFg_calculated values calculated to be less than 0. Minimum result: -2. Number of values below -5: 0. Number of NA results: 2. Please rerun the function with NegativeValueDF = TRUE if you wish to inspect these values.
#>
#> Negative values set to 0, as per user input.
#>
#> ---------------------------
#
NegativeCarb_results
#> food_code food_name WATERg PROCNTg FAT_g_combined FIBTGg_combined ALCg
#> 4 F0004 Mushroom 25 15 16 15 15
#> 6 F0006 Tomato 35 28 33 2 2
#> NA <NA> <NA> NA NA NA NA NA
#> NA.1 <NA> <NA> NA NA NA NA NA
#> ASHg comments CHOAVLDFg_calculated
#> 4 15 With imaginary nutrient values -1
#> 6 2 And blanks -2
#> NA NA <NA> NA
#> NA.1 NA <NA> NA
# Only the out of bounds results are present, in their original form, for inspection.
#
#
# The sixth example is of the CHOAVLDFg_calculator working on a dataframe with
# non-standard column names. It uses a modified example data frame, shown below.
#
breakfast_df_nonstandard <- breakfast_df_nonstandard[,c("food_code",
"food_name", "Water_values_g", "PROCNT_values_g", "FAT_values_g_combined",
"FIBTG_values_g_combined", "ALC_values_g", "ASH_values_g", "comments_column")]
breakfast_df_nonstandard
#> food_code food_name Water_values_g PROCNT_values_g
#> 1 F0001 Bacon 10 15
#> 2 F0002 Beans 15 10
#> 3 F0003 Toast 20 20
#> 4 F0004 Mushroom 25 15
#> 5 F0005 Eggs 30 21
#> 6 F0006 Tomato 35 28
#> 7 F0007 Sausage 40 10
#> 8 F0008 Butter NA 27
#> 9 F0009 Brown Sauce NA NA
#> 10 F0010 Tomato Ketchup NA NA
#> FAT_values_g_combined FIBTG_values_g_combined ALC_values_g ASH_values_g
#> 1 21 12 12 12
#> 2 12 3 43 3
#> 3 NA 8 8 28
#> 4 16 15 15 15
#> 5 11 6 6 6
#> 6 33 2 2 2
#> 7 13 9 9 9
#> 8 16 13 13 13
#> 9 NA NA NA NA
#> 10 NA NA NA NA
#> comments_column
#> 1
#> 2 These are imaginary food items
#> 3 <NA>
#> 4 With imaginary nutrient values
#> 5
#> 6 And blanks
#> 7 <NA>
#> 8 To test different outputs
#> 9
#> 10 And scenarios
# Notice how the column names are different, and differ from the assumed names.
#
#
# Because of the different names, the column names for each input must be specified.
nothing_results_NonStandardInput <- CHOAVLDFg_calculator(
breakfast_df_nonstandard,
WATERg_column = "Water_values_g",
PROCNTg_column = "PROCNT_values_g",
FAT_g_combined_column = "FAT_values_g_combined",
FIBTGg_combined_column = "FIBTG_values_g_combined",
ALCg_column = "ALC_values_g",
ASHg_column = "ASH_values_g",
comment_col = "comments_column"
)
#> ---------------------------
#>
#> 4 CHOAVLDFg_calculated values calculated to be less than 0. Minimum result: -2. Number of values below -5: 0. Number of NA results: 2. Please rerun the function with NegativeValueDF = TRUE if you wish to inspect these values.
#>
#> Negative values set to 0, as per user input.
#>
#> ---------------------------
nothing_results_NonStandardInput
#> food_code food_name Water_values_g PROCNT_values_g
#> 1 F0001 Bacon 10 15
#> 2 F0002 Beans 15 10
#> 3 F0003 Toast 20 20
#> 4 F0004 Mushroom 25 15
#> 5 F0005 Eggs 30 21
#> 6 F0006 Tomato 35 28
#> 7 F0007 Sausage 40 10
#> 8 F0008 Butter NA 27
#> 9 F0009 Brown Sauce NA NA
#> 10 F0010 Tomato Ketchup NA NA
#> FAT_values_g_combined FIBTG_values_g_combined ALC_values_g ASH_values_g
#> 1 21 12 12 12
#> 2 12 3 43 3
#> 3 NA 8 8 28
#> 4 16 15 15 15
#> 5 11 6 6 6
#> 6 33 2 2 2
#> 7 13 9 9 9
#> 8 16 13 13 13
#> 9 NA NA NA NA
#> 10 NA NA NA NA
#> comments_column
#> 1 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 2 These are imaginary food items; CHOAVLDFg_calculated calculated from 100-[constituents]
#> 3 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 4 With imaginary nutrient values; CHOAVLDFg_calculated calculated from 100-[constituents] - Original value of -1 reset to 0
#> 5 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 6 And blanks; CHOAVLDFg_calculated calculated from 100-[constituents] - Original value of -2 reset to 0
#> 7 CHOAVLDFg_calculated calculated from 100-[constituents]
#> 8 To test different outputs; CHOAVLDFg_calculated calculated from 100-[constituents]
#> 9
#> 10 And scenarios
#> CHOAVLDFg_calculated
#> 1 18
#> 2 14
#> 3 16
#> 4 0
#> 5 20
#> 6 0
#> 7 10
#> 8 18
#> 9 NA
#> 10 NA
#
# The resulting CHOAVLDFg_calculated column is the same as in the first example,
# despite the different names.